nuxt-oidc-auth
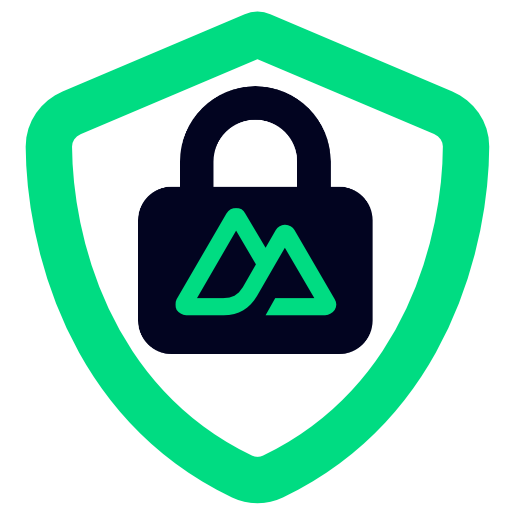
Nuxt OIDC Auth
Welcome to Nuxt OIDC Auth, a Nuxt module focusing on native OIDC (OpenID Connect) based authentication for Nuxt with a high level of customizability and security for SSR applications.
This module doesn't use any external dependencies outside of the unjs ecosystem except for token validation (the well known and tested jose
library for JWT interactions).
Features
↩️ Automatic session and token renewal
💾 Encrypted server side refresh/access token storage powered by Nitro storage
🔑 Token validation
🔒 Secured & sealed cookies sessions
⚙️ Presets for popular OIDC providers
📤 Global middleware with automatic redirection to default provider or a custom login page (see playground)
👤 useOidcAuth
composable for getting the user information, logging in and out, refetching the current session and triggering a token refresh
🗂️ Multi provider support with auto registered routes (/auth/<provider>/login
, /auth/<provider>/logout
, /auth/<provider>/callback
)
📝 Generic spec OpenID compatible connect provider with fully configurable OIDC flow (state, nonce, PKCE, token request, ...)
🕙 Session expiration check
Installation
nuxt-oidc-auth
dependency to your project Add
With nuxi
pnpm dlx nuxi@latest module add nuxt-oidc-auth
or manually
pnpm add -D nuxt-oidc-auth
Add nuxt-oidc-auth
to the modules
section of nuxt.config.ts
export default defineNuxtConfig({
modules: [
'nuxt-oidc-auth'
]
})
⚠️ Disclaimer
This module is still in development, feedback and contributions are welcome! Use at your own risk.